Taking SystemVerilog Arrays to the Next Dimension
In this session, you will learn the various array types in the SystemVerilog language, and how to pick the right ones for your testbench. As a result, your testbench code will be easier to understand and reuse, run faster, and consume less memory.
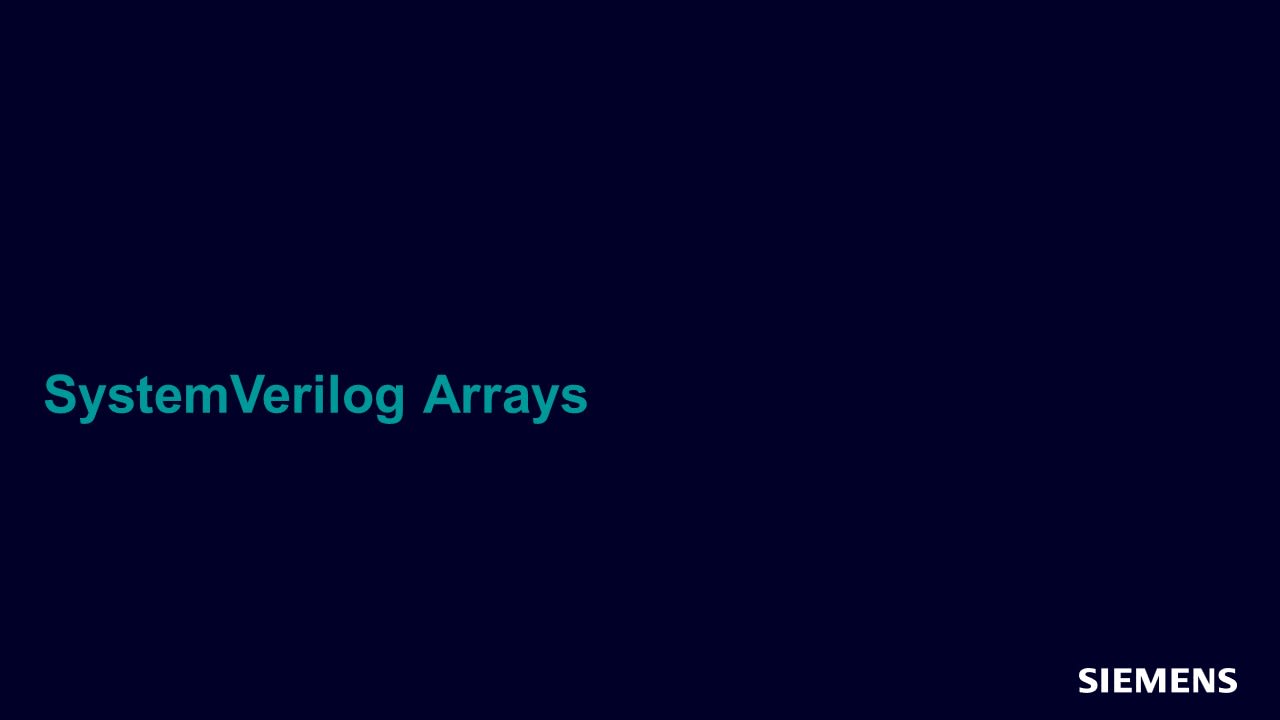
Full-access members only
Register your account to view Taking SystemVerilog Arrays to the Next Dimension
Full-access members gain access to our free tools and training, including our full library of articles, recorded sessions, seminars, papers, learning tracks, in-depth verification cookbooks, and more.